728x90
반응형
AES 란?
Advanced Encryption Standard의 약어로 직역하면 '고급 암호화 표준'이다. 일방향 대칭키를 쓰는 블럭 암호이다. 높은 안정성과 속도로 많이 사용되고 있는 암호화 알고리즘이다.
AES-256 암호화의 장단점?
비밀키 하나로 데이터를 암호화 / 복호화 한다. 장점으로는 보안성과 안정성이 높으며 속도가 빠르다. 단점으로는 키 한개로 암호화 / 복호화를 하기 때문에 키가 유출되는 경우 암호화의 의미가 없어진다.
AES-256 사용방법
AES-256 암호화 알고리즘을 사용하기 위해서는 3개의 jar 파일을 libraries에 추가해야 한다.
local_policy.jar
0.00MB
commons-codec-1.10.jar
0.27MB
US_export_policy.jar
0.00MB
AES256Cipher.java
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.InvalidAlgorithmParameterException;
import org.apache.commons.codec.binary.Base64;
public class AES256Cipher {
private static volatile AES256Cipher INSTANCE;
final static String secretKey = "dhtkdwnsdi199503291045dhtkdwns12"; //32bit
static String IV = ""; //16bit
public static AES256Cipher getInstance() {
if (INSTANCE == null) {
synchronized (AES256Cipher.class) {
if (INSTANCE == null)
INSTANCE = new AES256Cipher();
}
}
return INSTANCE;
}
private AES256Cipher() {
IV = secretKey.substring(0, 16);
}
//암호화
public static String AES_Encode(String str) throws java.io.UnsupportedEncodingException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
byte[] keyData = secretKey.getBytes();
SecretKey secureKey = new SecretKeySpec(keyData, "AES");
Cipher c = Cipher.getInstance("AES/CBC/PKCS5Padding");
c.init(Cipher.ENCRYPT_MODE, secureKey, new IvParameterSpec(IV.getBytes()));
byte[] encrypted = c.doFinal(str.getBytes("UTF-8"));
String enStr = new String(Base64.encodeBase64(encrypted));
return enStr;
}
//복호화
public static String AES_Decode(String str) throws java.io.UnsupportedEncodingException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
byte[] keyData = secretKey.getBytes();
SecretKey secureKey = new SecretKeySpec(keyData, "AES");
Cipher c = Cipher.getInstance("AES/CBC/PKCS5Padding");
c.init(Cipher.DECRYPT_MODE, secureKey, new IvParameterSpec(IV.getBytes("UTF-8")));
byte[] byteStr = Base64.decodeBase64(str.getBytes());
return new String(c.doFinal(byteStr), "UTF-8");
}
}
AES256CipherTest.java
package helloring.admin.util;
import java.io.UnsupportedEncodingException;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
public class AES256CipherTest {
static String id = "ohdaldal";
static String custrnmNo = "01012345678";
static String custNm = "오달달";
public static void main(String[] args) {
try {
AES256Cipher a256 = AES256Cipher.getInstance();
String enId = a256.AES_Encode(id);
String enYyyymmdd = a256.AES_Encode(custrnmNo);
String enCustNm = a256.AES_Encode(custNm);
String desId = a256.AES_Decode(enId);
String desYyyymmdd = a256.AES_Decode(enYyyymmdd);
String desCustNm = a256.AES_Decode(enCustNm);
System.out.println("id : " + id + " enId : " + enId + " desId : " + desId);
System.out.println("custrnmNo : " + custrnmNo + " enYyyymmdd : " + enYyyymmdd + " desYyyymmdd : " + desYyyymmdd);
System.out.println("custNm : " + custNm + " enCustNm : " + enCustNm + " desCustNm : " + desCustNm);
} catch (Exception e) {
// TODO: handle exception
}
}
}
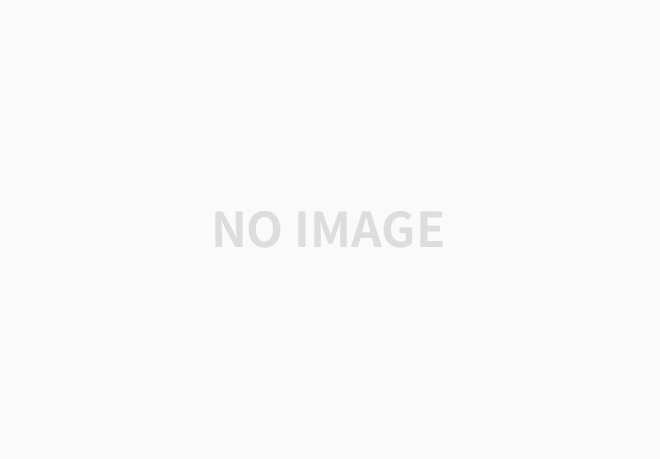
출처
http://jmlim.github.io/java/2018/12/06/java-aes256/
728x90
반응형
'IT > WEB' 카테고리의 다른 글
[JAVA] 파일 복호화(aes256)_참고용 (0) | 2021.04.16 |
---|---|
[JAVA] 파일 암호화(aes256)_참고용 (0) | 2021.04.16 |
[JAVA] 에러 java.lang.NoSuchMethodError 에러 메세지가 뜰 때.. (0) | 2021.03.12 |
[Eclipse] An Exception has been caught while processing the refactoring 에러 (0) | 2021.03.11 |
[JAVA] MySQL java.sql.Date 이슈(DATE 타입의 시분초가 나오지 않을 때)_java.sql.TimeStamp (0) | 2021.02.19 |